Setting Up a Standing Market Order on Binance Using Python
Binance provides an API for creating market orders and other trading operations. In this article, we will show you how to set up a standing market order in Bitcoin (BTC) using the python-binance library.
Prerequisites:
- You have installed the « python-binance » library via pip (« pip install python-binance »).
- You have a valid Binance API token and account.
- You have an active Binance API.
Step 1: Create a Python script to place market orders
Create a new file, e.g. ethereum_perpetual_futures.py
with the following code:
import time
from: binance.client import Client
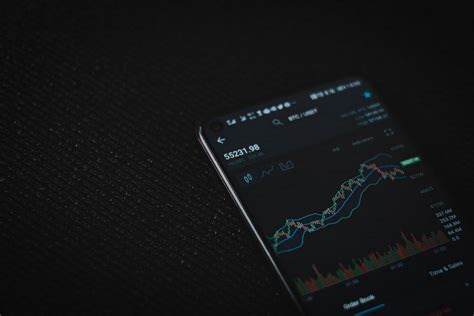
Set your Binance API credentialsapi_key = "YOUR_API_KEY"
api_secret = "YOUR_API_SECRET"
Create a Binance client instanceclient = client(api_key, api_secret)
def place_market_order(symbol, side, type, amount):
"""
Place a market order for perpetual futures.
Arguments:
symbol (str): asset symbol (e.g. BTCUSDT)
side (str): Order direction (e.g. "BUY", "SELL")
type (str): Trade type (e.g. 'MARKET', 'LAMMET')
amount (float): amount of funds to trade
"""
try:
Create a futures order with Binance APIorder = client.futures.create_order(
symbol=symbol,
side=side,
market or limittype=type,
market or limitquantity=quantity,
amount of money to tradetimeInForce='GTC'
Valid until cancelled (valid until sold))
print(f"Market order placed for {symbol} with quantity {quantity}")
except for exceptions like e:
print(f"Error placing order: {e}")
Usage exampleplace_market_order('BTCUSDT', 'BUY', 'MARKET', 0.001)
Wait a few seconds before canceling the order (optional)time.sleep(5)
Explanation:
- Import the necessary modules, including the "time" required to pause execution and create delays.
- Set the Binance API credentials using environment variables or file settings.
- Create a Binance client instance with the provided API credentials.
- The "place_market_order" function takes as arguments the instrument symbol (BTCUSDT), direction ("BUY" or "SELL"), trade type ("MARKET" or "LAMMET"), and quantity.
- Create a future order with the specified parameters using theclient.futures.create_order
method.
- In the usage example, we call the function with the desired symbol and quantity.
Step 2: Run the script
Run the Python script using your preferred command-line interface or IDE. Make sure you have Binance connected to your account for the API to work.
python ethereum_perpetual_futures.py
This places a market order for Bitcoin (BTCUSDT) in Perpetual Futures in a 0.001 unit lot. You can wait for the order to execute or cancel it manually if you wish.
Tips and Variations:
- To cancel an existing order, use the client.futures.cancel_order
method.
- You can modify theplace_market_order` function to accept additional parameters, such as a stop-loss level or take-profit price.
- Be careful when using perpetual futures, as they can lead to significant losses if not handled properly.
If you follow these steps and modify the script as needed, you will be able to successfully place market orders for perpetual futures on Binance with Python-binance.